Below you will find pages that utilize the taxonomy term “Security”
The terrible random number generation in the Commodore 64 (and 128)
Quite a while ago, I started playing with random numbers on 8 Bit machines. I don’t think anyone is doing “serious” work on these machines, but playing with Ciphers and Crypto got me at least curious about how a Commodore 64 generates random numbers.
There are many ways to determine randomness. Humans are pretty good at picking out patterns in visual representations. Luckily our beloved machines have easy screen memory access. Let’s poke some random stuff to the screen and see what we see.
Making and breaking Ciphers on the Commodore 64, er VIC-20 - Lagged Fibonacci Sequence and a little Monte Carlo while embracing contraints
We’re on ANOTHER random number generation technique today. Let’s work on the Lagged Fibonacci Sequence, a fun way to use the Fibonacci Sequence on itself to generate pseudo-random numbers.
One of our long term goals is to add a bunch of tools to our toolbox. Making reusable components makes doing more complicated things easier. You can rifle through your toolbox in hopes of an “Oh, I already have something we can use for this part!”.
Making and breaking Ciphers on the Commodore 64 Part 12 - Pontifex - Solitaire from Cryptonomicon
Cryptonomicon Pontifex/Solitaire
We’re back to my favorite book for another round. We’ve done quite a bit with Cryptonomicon so far. One of my favorite moments is when Enoch Root introduces a cipher that two prisoners used to communicate using just a humble playing card deck.
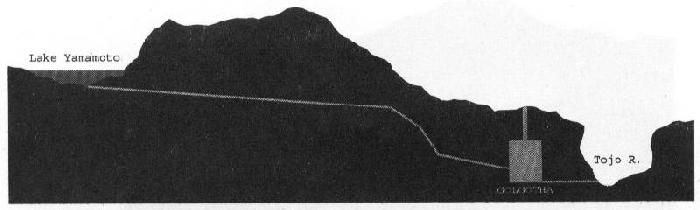
Drawing of the mine in the book
Bruce Schneier
Pontifex is an encryption method you can do with another person using a deck of cards. It was invented for “Cryptonomicon” for Neal Stephenson by Bruce Schneier. He called it Solitaire. In the book it’s called Pontifex to obfuscate the method a little bit.
Making and breaking Ciphers on the Commodore 64 Part 11 - One time pads on a Commodore 64, probably a bad idea
A while ago, I explained in detail how to generate one time pad sheets and use them to encrypt and decrypt messages.
It turned out to be doable, only requiring a pencil and a way to create random numbers, but it’s slow, error prone and tedious.
Why
The one time pad article was born out of my annual reading of Cryptonomicon. I wanted to learn how to make these pads, which if used correctly are mathematically unbreakable. I figured 3 or 5 other people might read it and learn how to make them too.
Making and breaking Ciphers on the Commodore 64 Part 10 - Finding hash collisions with a type in game from 1984
Hashes
We did an implementation of a once popular hashing function in Part 8 on RC4 without really going into why.
A hash function is any function that is used to map data of an arbitrary size (input) to a fixed size output. An algorithm is applied to the input and results in a fixed length output called the hash.
In cryptography it’s important to get a repeatable output for a given input, but ideally NO information about the input that created it.
Making and Breaking Ciphers with Commodore 64 Part 9 - Finding Smallish Primes
Recap
So far we’ve
- Reversed a string
- Shifted bits left and right (mostly right)
- Brute forced a known simple cipher
- Channeled our inner John Connor by cracking ATM machines
- Searched through a large number looking for matches
- Learned how to use XOR to recover data
- Created a Linear Feedback Shift Register to generate better random numbers
- Implemented a “real” but outdated cipher: RC4
Disclaimer: I’m sure I’ll get an angry email from a mathematician about this, but we’re going for understanding here. No one expects to be looking for large primes on a Commodore 64. Calm down.
Making and breaking Ciphers on the Commodore 64 Part 8 - RC4
Recap
So far in this 8 part series on doing weird things with Ciphers on old computers we’ve:
- Reversed a string
- Shifted bits left and right (mostly right)
- Brute forced a known simple cipher
- Channeled our inner John Connor by cracking ATM machines
- Searched through a large number looking for matches
- Learned how to use XOR to recover data
- Created a Linear Feedback Shift Register to generate better random numbers
The warning
“In September, 1994 someone posted source code to the Cyberpunks mailing list-anonymously. It quickly spread to the Usenet newsgroup sci.crypt, and via the Internet to ftp sites around the world. Readers with legal copies of RC4 confirmed compatibility. RDA Data Security, Inc. tried to put the genie back into the bottle, claiming that it was still a trade secret even through it was public, it was too late. It has since been discussed and dissected on Usenet, distributed at conferences, and taught in cryptography courses.” - Applied Cryptography, Bruce Schneier, 1997
Making and breaking Ciphers on the Commodore 64 Part 7 - Pseudo Random with Linear Congruential Generators
So far in this 7 part (shocked I’ve made it through 7!) series on doing weird things with Ciphers on old computers we’ve:
- Reversed a string
- Shifted bits left and right (mostly right)
- Brute forced a known simple cipher
- Channeled our inner John Connor by cracking ATM machines
- Searched through a large number looking for matches
- Learned how to use XOR to recover data
Author’s Note:
This one stretched my skills quite a bit, but I kept it under 2000 words (by 89). I’m not sure when I started this I knew I’d be doing a Linear Congruential Generator on a 40 year old machine, but here we are ;-)
Making and Breaking Ciphers with a Commodore 64 - Part 6: XOR is Magical - Data recovery
Review
So far we’ve:
- Reversed a string
- Shifted bits left and right (mostly right)
- Brute forced a known simple cipher
- Faked it, but then looking for a 4 digit known pin
- Searched through a large number looking for matches
We now have most of the basic skills in Assembly to start doing some real work. Let’s tackle what is arguably the most important of the bitwise operators for ciphers and encryption: The exclusive OR. (XOR/EOR)
Quick post: Determining length in Commodore Assembly
A common task in our code is to determine the length of something. In this example, let’s check the length of a string like “are you keeping up?”
In Python, this is crazy easy.
#!/usr/bin/env python
message = "are you keeping up?"
len(message)
In 6510 Assembly, this is also easy. Not crazy easy, but straightforward anyway.
messagelength
ldx #$00
checkdel
lda message,x
cmp #$00 ; or whatever we're using as a delimeter
beq done
inx
jmp checkdel
done
stx messagelen
rts
.byte messagelen 0
message .null "are you keeping up?"
Pretty easy right?
Making and Breaking Ciphers with a Commodore 64 - Part 5: Wargames
Let’s recap a little
So far we’ve:
- Reversed a string
- Shifted bits left and right (mostly right)
- Brute forced a known simple cipher
- Faked it, but then looking for a 4 digit known pin
What we need next in our toolbox is to be able to search through a large number of values to look for something. This will build on the previous skills and also reinforce working with tables and 24 bit math on an 8Bit Processor.
Making and Breaking Ciphers with a Commodore 64 - Part 4: The PIN Program from Terminator 2
A few minutes into Terminator 2, the young John Connor takes his Atari Portfolio out of his bag and inserts a foil covered card into an ATM machine and proceeds to crack a pin number and steal some cash from an unsuspecting account.

This is a movie prop and doesn’t really appear to do anything useful. It’s almost certainly just a script that runs on the Atari Portfolio, and just counts down some strings to make a “cool” effect.
Making and Breaking Ciphers with a Commodore 64 - Part 3: The Caesar Cipher
Well here we are at part three and it’s a lot to pack into one page.
Let’s build upon [what I called the Shift Cipher] and implement what is often referred to as the Caesar Cipher, although there is much debate on when this was really first done.
The idea is the same as our Part 2. We’re going to shift the letters a given number of times, but this time we’re going to do the encoding and decoding programmatically instead of just creating a reference to do it by hand.
Making and Breaking Ciphers with Python, er Commodore- Part 2: The Shift Cipher
In Part 1 we did a simple reverse of a string. While simple, these software steps will help build the skills we need going forward in Assembly without feeling like I skipped right to a 300 line after doing a short one. One foot in front of the other and all that.
The Shift Cipher
For Christmas, my wife and I bought my sister’s kids this code wheel. It implements a simple shift cipher to be transposed by hand. This was common low tech way to scramble messages for quite some time, and fits nicely in between reversing a string and a more complex implementation of the Caesar cipher in software.
Making and Breaking Ciphers with Python, er, a Commodore- Part 1: The Reverse Cipher
Sometime during my weeklong exploration of SHA-256 on a Commodore realized that there was a whole world of exploration that could be explored. Just because a Computer system is old, doesn’t mean it’s not interesting or useful.
I had expected it to take months to figure out the SHA-256 implementation, but it only took me a week or so. This was pretty surprising to me and is an excellent demonstration of what might be possible on a Commodore 64
Simple, 'unbreakable' encryption with a pencil
Last week, at this bat-time on this bat-channel, I talked about checking off one of my 35 year old bucket list items by learning Commodore assembly language. (shut up, you’re old too.) Coincidentally, I picked this up at the time I was re-reading Cryptonomicon by Neal Stephenson for the ump-teenth time. There are a few books that I consider so good that they’re worth re-visiting, and this is one of them.
If you haven’t read it, you don’t need to worry about spoilers. The plot is multi-generational. One character, Lawrence Waterhouse, is a high level Allied Cryptographer tasked with cracking codes and coming up with new encryption methodologies. His weapon of choice for the most secure messages is the one time pad.